So you had mentioned windows 3.1 before, do you still have a 3.1 original machine.
As everything I'd run under windows 3.1 runs pretty much flawless under 9x, I've got a Win 98SE rig.
AMD 5x86-133 (fits a 486 socket) "overclocked" to 150mhz. It's technically NOT an overclock as it's within the design spec, but it's not something they advertised because a lot of 486 motherboards didn't support the real DX50 with its 50mhz base clock. The vast magority of machines could only support 25 or 33mhz, and in some cases 40mhz. Thus it was most common for these chips to go 133 (33x4) or 120 (40x3) or 100 (25x4).. but it does support the 3x modifier as well to push a 50mhz base clock to 150mhz.
Was one of the first CPU's to ever come with a heatsink and fan in the box instead of as a separate purchase.
It's funny though, as 3x 40mhz for 120mhz CPU clock was often faster than 4x33mhz at 133mhz CPU because of the faster data bus. At 133 it was roughly equal to a pentium 75. At 150mhz 3x50, it gives a Pentium 90 a run for its money... and is even faster in some cases. For example Diablo REALLY needs a pentium 120 to be enjoyable, but you can run it on a AMD 5x86 at 150mhz quite reasonably despite the huge IPC disparity.
The mobo for that machine in question:
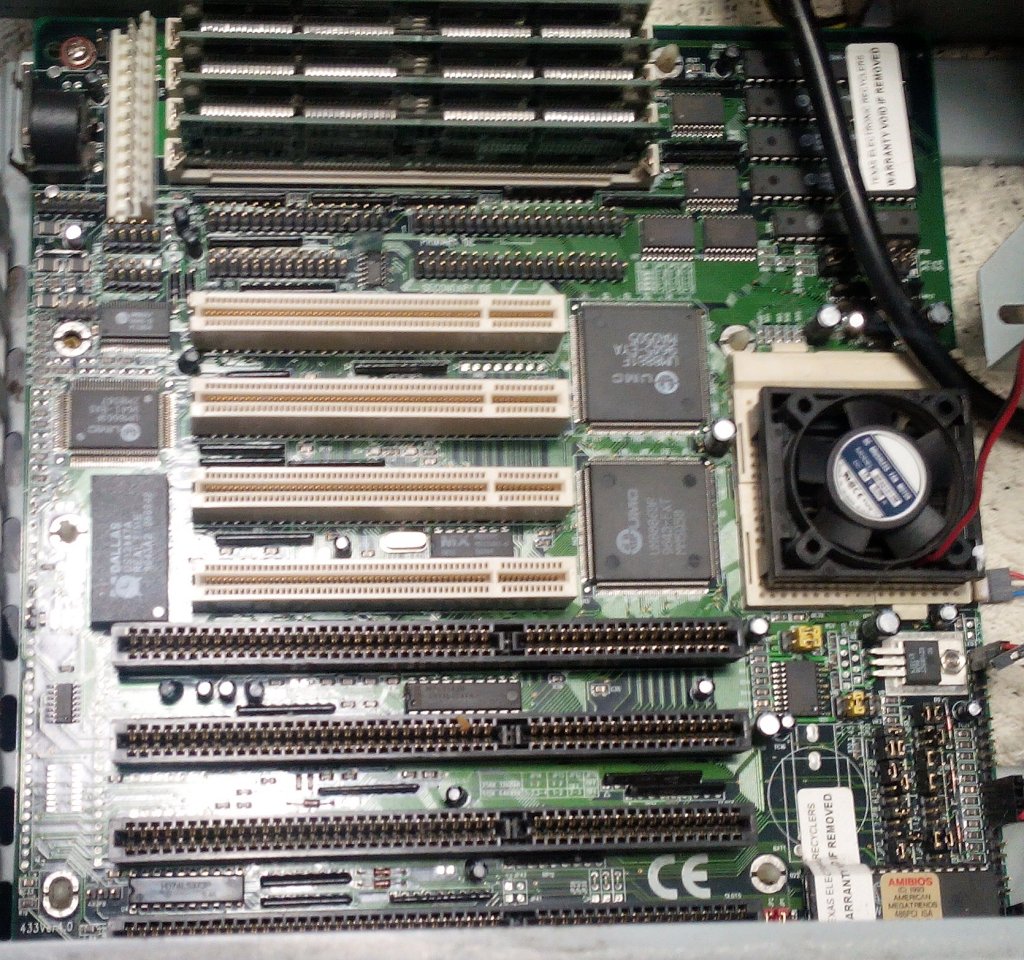
486 class board, but nice because it has PCI, supports large denisity EDO memory, has two 32 bit IDE ports and PS/2 mouse built in (the five pin connector near the keyboard, takes a dongle), etc, etc.
That's 128 megs of 32 bit EDO memory sitting in there. It's comedy watching it start up trying to count up to that... and the BIOS has no memory test skip. Power on the machine, go get coffee... at Dunkins... the next town over... and take your time.
It's also a bit amazing for the time as it has half a megabyte of SRAM cache for the CPU. Compared to normal 486 boards that had either 64 or 128k of SRAM, the massive cache nets you around another 10% performance in many tasks.
I paired it with a PCI voodoo 2 and a Trident 4000 card.
I suppose anyone today with a 3.1 machine with a whopping 100MB hard drive would be paid a good bit of money for it.
You'd be surprised. Since 9x is basically fully 3.1 compatible the window to hardware is a lot bigger. Fans also tend to make machines that use compact-flash to replace the aging hard drives.
Even my tired old Tandy 1000SX is booting DOS 6.22 off a 128mb CF card, via an XT-IDE controller.
The XT-IDE project is tons of fun, I ordered mine as a kit in the form of the version 3, so I had to solder everything onto the board.
The IDE / PATA interface -- at least in its original incarnation -- is something of a joke as apart from having only 3 address lines (so you needed an encoder to sit between the plug and the bus to decode addresses) it was otherwise a direct mapping of the 16 bit ISA bus with all 16 data lines. Quite literally apart from an address decoder, all the wires from the drive went straight to the system bus. It's kind of brilliant as a little software on the CPU to give directions, a hold line to tell the CPU "hang on, I'm writing to memory" and putting the drive controller on the drive itself, and boom, the controller card in the machine was really simple.
The problem is on old PC/XT class systems you don't have a 16 bit bus, you have a 8 bit bus.
As such to use conventional 16 bit IDE drives on a PC or XT class machine (8088 / 8086) you need the controller to buffer the results from the drive. The controller wants to read 16 bits, you have to tell it "hold on", have an expansion card do the two reads required, then output them to the drive. Same process for a write where the disk's board will try to write 16 bits to memory, and your controller has to cache that and write it out in two operations.
Hence the XT-IDE controller was born. Laugh is in some cases because of the ability to buffer and the more modern software written for it, and by switching the drive to "polling" instead of "bus mastering" mode, it's possible for the XT-IDE to be faster than flat IDE in some 286 class machines. Mostly that's not so much the hardware's fault, as that of poorly written BIOS.
Which is why there's a version of the XT-IDE BIOS you can burn to ROM and use on 286's. Uses the normal hardware, but speeds things up just because the code and methodology isn't shite.
Even so, even without optimizations a modern CF card -- which itself is just a IDE device with a different plug -- will run circles around period correct hard drives. (no seek times). Basically, it's SSD's for vintage PC's.
Though I have gotten a 16 gig SATA SSD to recognize on XT-IDE in my T1k via a SATA to PATA adapter. The only issue is that there's no OS I can run on it that will recognize a Hard Drive that large.
I do wish keyboards today would last as long as those old ones did. This weekend i took all the keys off my gaming keyboard and cleaned under them... it was gross man after 6 years lol..... But 3 of the key dont even have the letters on them anymore. Those old keyboards were like old cars, built to last...
You can still get decent keys, but yeah... what these keyboards ship with is junk. The "Aurealor Roamer" I have on my media center came with some lousy painted keys that yeah, you can wear the text right off of, but that's why I suggest double-shot aftermarket keys. For a reasonable price off of e-bay you can usually grab a full set for around $15 or less. So long as the keyboard in question uses cherry-style switches -- so that includes Gateron, outemu, etc -- they'll fit as it's pretty well standardized.
I actually often order three or four sets at a time to do custom colour layouts... for fun, for friends, and even for profit. You take a cheap-ass mechanical keyboard, replace the caps with decent stuff, add some of the aftermarket rubber o-rings to soften how they bottom-out, and you're good to go.
I still have some old 3.5 inch discs somewhere with some 3.1 games on them, and i have 1 5.25 inch floppy that i just keep in a old box to show my kids the way it used to be done.
You'd laugh at my collection -- the MAC SE that can only read their proprietary 800k 3.5" format, the Commodore 64 with TWO working 5.25" floppy drives, The Coco 1 and Coco 3 with their 160k single sided floppies... even my Tandy 1000 and PCJr only supports 360k 5.25" floppies.
Which is part of where that AMD5x86 win9x box comes into play. XP/later really hates or even outright doesn't support low density floppies well if at all. Some newer motherboards even if they have floppy connectors don't support 5.25"... so that 98SE box serves double duty for retrogaming/retrocomputing, and being a "middling machine"
Middling machines is the term for systems that are just old enough to support old media formats, but just new enough to talk to modern machines. I can get it on the LAN, I can get it to talk to my NAS... so I can use it to read my modern work and copy stuff to the old media. Because it has a parallel port, I can even use it to write to the Commodore 64 floppy drives using an adapter.
(said drives being utterly incompatible with PC standard drive formats)In fact in writing Paku Paku, most of the development was done in Flo's notepad 2, compiling the assembly with NASM native under Win7, but then the Turbo Pascal parts compiled and tested under DOSBox. To test it on the real iron, I uploaded to the NAS, downloaded to the 98 rig, then wrote to the old-school floppies.
I think i remember space shooter
I said "A space shooter" -- as in the generic category. Actual games of the genre includes Space Invaders, Galaxian, Galaga, Dorf, and the like.
i still think astroids was amazing for its time, all the rolling and spinning of the astroids and the ship which if i remember was not much more than a colored in "A" and the movements if you remember were cool when you bounced off stuff,
It was also a vector graphics game, which means it ports poorly to raster graphics computers.
Instead of a constant sweep breaking the screen into separate pixels, a vector graphics display literally moves the electron stream from point to point, turning the beam on and off like a mechanical plotter. That's why the lines are smooth is there's no colour mask or scanlines involved. Each and every line is drawn directly, not as a bunch of separate pixels.
Which is why the Atari 2600 version is utter garbage:
https://www.youtube.com/watch?v=xP1Jtjk5vXYAnd that's with twice the display resolution available that I'm using. Even the 5200 version which was a far more capable machine:
https://www.youtube.com/watch?v=KCL_GKJVS2YIs pretty shite...
In fact, pretty much all the computer ports are trash.
https://www.youtube.com/watch?v=UqghsUAzBsQAt least until resolutions started climbing up past 320x200.
Vector graphics were short lived, and it's kind of a shame. All the games made with it -- Asteroids, Omega Race, Tempest, Star Wars, Star Trek -- had a very distinct look and feel. Porting any of them to raster displays was always junk regardless of platform.
I mean hell, look at the Star Trek Arcade Game:
https://www.youtube.com/watch?v=GuV-iqqrT4YAnd the Atari 5200 port:
https://www.youtube.com/watch?v=zgvo8OWhT34Which is sad since the 5200 is from a purely hardware standpoint MORE capable than the Star Wars arcade. But the 5200 was limited by having to display on a TV using raster graphics.
Funny thing is, there was actually a game console -- that had it's own screen -- called the Vectrex that was all vector graphics.